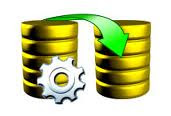
controller.newRecord();This will create a new record as the first row, and will update the user interface. In most cases this is OK - however, there may be times when you want to add records and not update the user interface. In this case you want to use the foundset object:
myField = "value";
foundset.newRecord();Now that's all find and good - the record will be created and the user interface won't immediately change (until you call databaseManager.saveData()). This method is much faster when you're creating a bunch of records (or related records).
foundset.myField = "value";
Now, something that I use when I'm using the second method is to grab a record object reference when I create the record - so I can choose to commit that newly created record right away. Here's the slightly modified code:
var record = foundset.getRecord(foundset.newRecord());Because we have a reference to the foundset record in the variable called "record" - we can tell the databaseManager to only save that one record (rather than all outstanding changes).
record.myField = "value";
databaseManager.saveData(record);
2 comments:
I actually load the following utility functions in my solutions on a $fs global because these things come up so often it saves some typing:
// foundset convenience methods
%fs = { };
// return current record from foundset
$fs.rec = function(fs) {
return fs.getRecord(fs.getSelectedIndex());
};
// return new record in foundset
$fs.new = function(fs) {
return fs.getRecord(fs.newRecord());
}
AWESOME TIP, Greg! Thanks very much for sharing!
BTW: If you don't already know - Greg is the author of the FABULOUS Terminology application for iPhone and iPad - I highly recommend them!
Post a Comment